python 编码规范
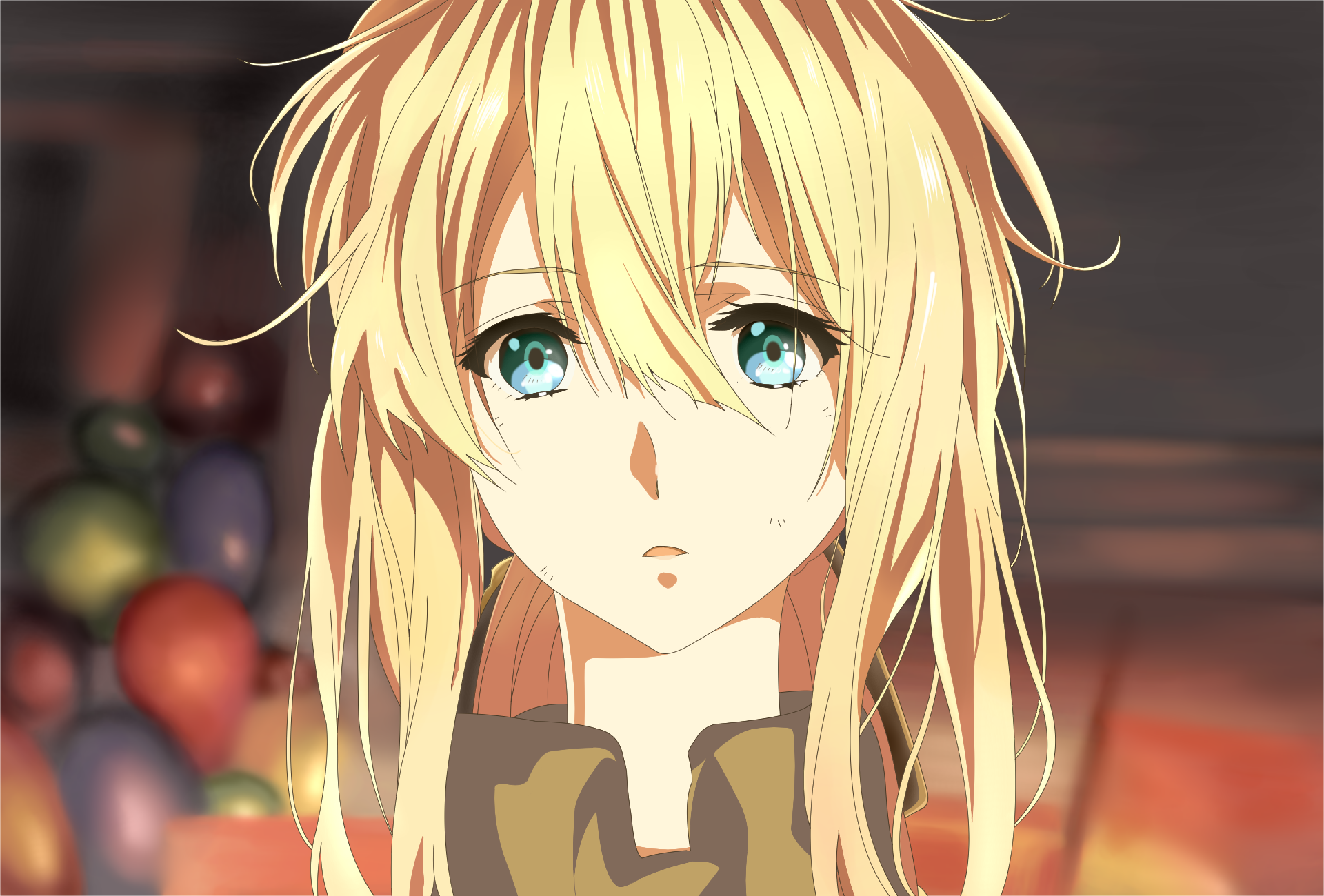
编码
- 无特殊情况,文件一律使用 UTF-8 编码
- 无特殊情况,文件头部必须加入
# -*- coding: utf-8 -*-
缩进
- 统一使用 4 个空格进行缩进
引号
- 自然语言,使用双引号。例如
"hello world"
- 机器标识,使用单引号。
- 正则表达式,使用双引号。
- 文档字符串,使用三引号。
空行
- 模块级函数和类定义之间空两行
- 类成员函数之间空一行
- 函数中可以使用空行分隔出逻辑相关的代码
1 | class A: |
import 语句
- import 应该分行书写
- import 应该使用 absolute import
- import 放在文件头部,置于模块说明及
docstring
之后,与全局变量之前docstring
- import
- 全局变量
空格
- 二元运算符两边
- 函数的参数列表
,
之后要有空格 - 函数的参数列表中,默认值等号两边不要加空格
- 括号不需要加空格,字典类似
- 不要添加多余空格,用于对齐
=
1 | def foo(a, b=10): |
换行
python 支持括号内的换行,第二行缩进到括号的起始处,参数对齐
1 | bar = foo(a1, a2, |
使用反斜杠\换行,二元运算符+ .等应出现在行末;长字符串也可以用此法换行
1 | session.query(MyTable).\ |
if / for / while 独占一行
Docstring
作为文档的 Docstring 一般出现在模块的头部、函数和类的头部,这样在 python 中可以通过对象的 __doc__
对象获取文档。编辑器和 IDE 也可以根据 Docstring 给出自动提示。
- 所有的公共模块、函数、类、方法,都应该写
docstring
。私有方法不一定需要,def 后提供一个块注释来说明 - docstirng 的结束,应该独占一行,除非只有一行
1 | """Return a foobar |
Google 风格:
1 | # -*- coding: utf-8 -*- |
命名规范
模块
模块尽量使用小写命名,首字母保持小写,尽量不要使用下划线
1 | # 正确的模块名 |
类名
类名使用驼峰命名风格,首字母大写,私有类可用下划线开头。
1 | class Farm(): |
将相关的类和顶级函数放在同一个 module 里. 不像 Java , 没必要限制一个类一个 module.
函数
函数名一律小写,有多个单词,使用下划线隔开。私有函数在函数前加一个下划线。
1 | def run(): |
变量名
尽量使用小写,如有多个单词,使用下划线隔开
1 | if __name__ == '__main__': |
常量
使用全部大写
1 | MAX_CLIENT = 100 |
- Post title:python 编码规范
- Post author:auggie
- Create time:2022-04-11 15:07:06
- Post link:https://ruanjiancheng.github.io/2022/04/11/encode-python/
- Copyright Notice:All articles in this blog are licensed under BY-NC-SA unless stating additionally.