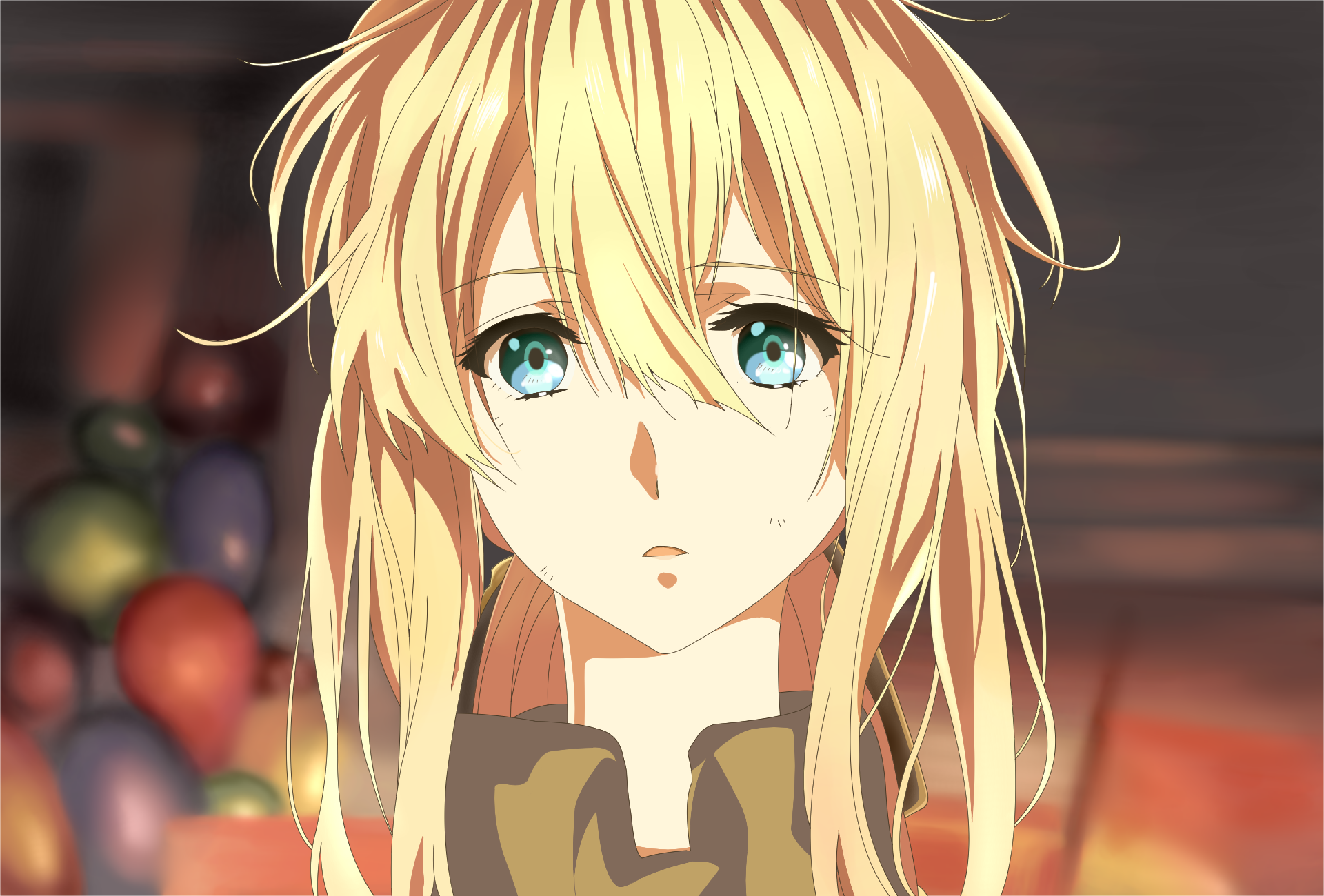
Vue
什么是 Vue
Vue.js(读音 /vjuː/, 类似于 view) 是一套构建用户界面的渐进式框架。
Vue 只关注视图层, 采用自底向上增量开发的设计。
Vue 的目标是通过尽可能简单的 API 实现响应的数据绑定和组合的视图组件。
1 | <script src="https://cdn.jsdelivr.net/npm/vue@2.6.14"></script> |
第一个 vue 程序
el 挂载点
Vue 实例的作用范围是什么?
在 el 命中的内部都可以使用
是否可以使用其他的选择器?
可以使用
- id 选择器 id=””,建议挂载在 div 下面
#app
- class 选择器
.app
- 标签选择器
- id 选择器 id=””,建议挂载在 div 下面
作用域
- 所有的双标签,除去 body,html
- 不持支单标签
data 数据对象
基础类型 string
1
message:"hello!"
数组 array
1
campus:["1", "2", "3"]
可以使用数组索引访问
对象 object
1
2
3
4school : {
name:"name",
mobile:"xxxx"
}支持全部渲染,也可以使用
.
访问
methods 属性
用于添加方法
本地应用
通过 vue 实现常见的网页效果
- 内容绑定、事件绑定
- 显示切换、属性绑定
- 列表循环,表单元素绑定
内容绑定、事件绑定
v-text
内容绑定
用于设置标签的内容
- 标签内部使用 v-text 语法,只能全部替换
- 使用插值表达式,支持部分替换
- 支持内部字符串拼接
1 | <div id="app"> |
v-html
内容绑定
与 v-text 语法类似,但是设置的字符串是 html 标签的时候,v-html 会被解析出来
1 | <div id="app"> |
v-on
事件绑定
- 传递兹定理参数
- 事件修饰符
容器:
1 | <div id="app"> |
vue 实例:
1 | <script> |
更改页面显示:
在事件中修改数据。
计数器案例:
1 |
|
显示切换、属性绑定
v-show
用于操作显示状态
根据表达式的真假,切换元素的显示和隐藏。
可以直接在 v-show=”” 中写表达式。
1 | <div id="app"> |
v-if
与 v-show 类似。
频繁切换的时候,使用 v-show,否则使用 v-if
1 | <div id="app"> |
v-bind
操作元素属性
列表循环,表单元素绑定
v-for
用于列表循环遍历。
(item, index) in DS
可以动态修改
v-model
data 同步获得表单信息
实例,记事本:
- v-for
- v-on
- v-model
- v-text
1 | <div id="note"> |
网络应用
vue 如何结合网络数据开发应用
axios 网络请求库
axios
功能强大的网络请求库
1 | <script src="https://unpkg.com/axios/dist/axios.min.js"></script> |
1 | https://autumnfish.cn/api/joke |
简单 axios + vue 程序
1 | methods: { |
CSS
CSS 的导入方式
在 html 中直接编写 style(内部样式)
1
2
3
4
5选择器 {
声明1:XXX;
声明2:XXX;
声明3:XXX;
}放在 CSS 文件夹中,引入 CSS
1
<link rel="stylesheet" href="CSS/style.css">
行内属性
1
<h1 style="color: cornflowerblue">css</h1>
优先级:就近原则
选择器
1. 基本选择器
标签选择器
1
2
3h1 {
color: blanchedalmond;
}类选择器
1
2
3.test {
color: aquamarine;
}id 选择器,id 全局唯一
1
2
3#test {
color: blueviolet;
}
不遵循就近原则,id > class > 标签
2. 层次选择器
后代选择器
1
2
3
4body p {
background: cornflowerblue;
border-radius: 3px;
}子选择器
1
2
3
4body > p {
background: cornflowerblue;
border-radius: 3px;
}兄弟选择器
选择下面一个兄弟
1
2
3
4
5
6<style>
.active + p {
background: cornflowerblue;
border-radius: 3px;
}
</style>通用选择器
当前标签下面的全部兄弟标签
1
2
3
4
5
6<style>
.active ~ p {
background: cornflowerblue;
border-radius: 3px;
}
</style>
3. 结构伪类选择器
- 选中 ul 的第一个子元素
- 选中 ul 的最后一个子元素
1 | ul li:first-child { |
4. 属性选择器
“value 是完整单词” 类型的比较符号: ~=, |=
“拼接字符串“ 类型的比较符号: ***=**, ^=, $=
1. attribute 属性中包含 value:
[attribute~=value] 属性中包含独立的单词为 value,例如:
1 | [title~=flower] --> <img src="/i/eg_tulip.jpg" title="tulip flower" /> |
[attribute*=value] 属性中做字符串拆分,只要能拆出来 value 这个词就行,例如:
1 | [title*=flower] --> <img src="/i/eg_tulip.jpg" title="ffffflowerrrrrr" /> |
2. attribute 属性以 value 开头:
[attribute|=value] 属性中必须是完整且唯一的单词,或者以 - 分隔开:,例如:
1 | [lang|=en] --> <p lang="en"> <p lang="en-us"> |
[attribute^=value] 属性的前几个字母是 value 就可以,例如:
1 | [lang^=en] --> <p lang="ennn"> |
3. attribute 属性以 value 结尾:
1 | [attribute$=value] 属性的后几个字母是 value 就可以,例如: |
美化网页元素
约定使用的标签名:
- span 标签:重点要突出的字,使用 span 标签套起来。
字体样式
1 | #font-test { |
文本样式
- 颜色
- 对齐方式
- 首行缩进
- 行高
- 装饰
1 | #text-test { |
文本 与 图片对齐
1 | img, span { |
总结
前端
创建文件时
- 使用 vue 开发。使用 vue ui 创建工程的时候,需要勾选两个服务 route 和它下面的一个。
- 需要添加 axios 插件,通过
vue add axios
来完成
编写文件时
- vue 是单文件项目,所有的文件都在一个 html 中,通过 ref-link 跳转到不同的 .vue 文件中,刷新页面
- 在 views 中编写页面之后,需要在 router 下面的 index.js 注册路由
- 在 app.vue 中,编写跳转标签
动态获取路由渲染 nav
- 通过
$router.options.routes
获取路由信息,通过v-for
即可获取当前项
前端处理表格
切换页面
- 自带属性有 page-size,total 等
- 切换页面可以通过 current-change 函数实现
表格
- 只会通过当前页面来渲染页面
前端表单处理
- 可以前端校验表单的合法性
- 直接向后端提交 axios 请求,通过 ,追加表单信息
- 成功后,跳转
修改页的处理
update 页面如何获取数据
- 旧页面通过
$router.push({path: 'url', query:{id: row.id}})
传递参数 - 新页面通过
this.$route.query.id
获取传递参数
- 旧页面通过
触发点击事件后,将 id 传到另一个页面
新页面通过 id 请求后台数据
知识点
- 添加
created(){}
函数可以在页面初始化的时候,刷新页面 - 动态刷新
windows.location.reload()
- 跳转
this.$router.push({path:'', query:{}})
一些错误
- axios 采用 https 链接报错,采用 http 链接
- axios 需要在函数外面保存对象,
const _this = this
后端
使用 springboot + mybatis 实现
- controller 需要添加
@Controller
注解 - service 需要添加
@Service
注解 - dao 需要添加
@Mapper @Repository
注解 - Test 需要给类添加
@SpringBootTest
和 函数添加@Test
注解
配置文件
application.yml
1 | spring: |
*Mapper.xml
1 |
|
controller 文件
1 | // 使用 restful 开发 |
后端处理表格
- 编写获取数据大小的接口
- 编写根据当前页和页面大小的数据接口,restful 风格获取
后端表单处理
- 通过 @RequsetBody 获取 JSON 格式的信息
- 然后处理即可
一些常犯的错误
- 没有启动 mysql
service mysqld start
- mybatis 通过对象传递参数的时候,需要在函数中添加 @Param 的注解,并且不需要在 xml 中配置 paramType
- Post title:前端基础学习笔记
- Post author:auggie
- Create time:2022-05-14 21:48:00
- Post link:https://ruanjiancheng.github.io/2022/05/14/html-css-vue/
- Copyright Notice:All articles in this blog are licensed under BY-NC-SA unless stating additionally.